Hey folks,
Today we will have a look at how to add Emoji into your SharePoint site script / site template. You might already notice, it’s easy to add them manually into your navigation, but it’s hard to add via Site Designs. Let’s get started.
What are SharePoint site templates and site scripts?
Let’s start with a brief overview of what you can use SharePoint site templates and scripts for. As you might suggest from the name, templates help you to easily apply the same look and feel on all sites with the same purpose. Like think about your department sites or project sites in your organization, you don’t want to start configuring all the lists, libraries, navigation elements, and branding repeatedly for every new project site that is created within your organization and even the users should be able to do this manually or it should happen automatically.
That is where SharePoint site templates come in.
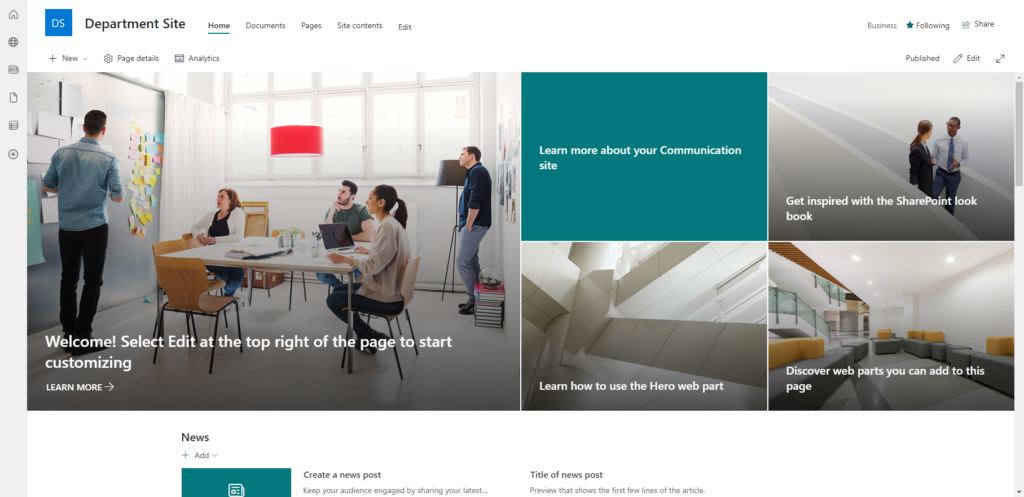
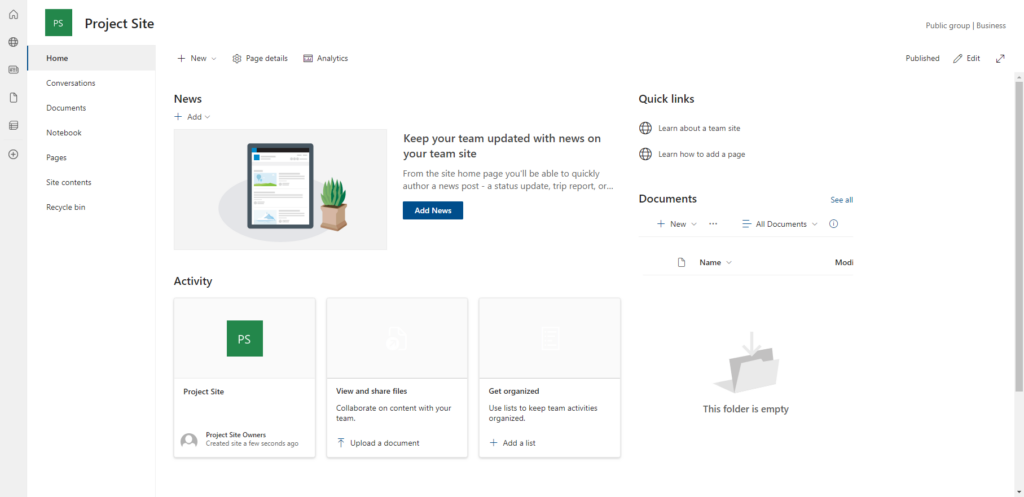
Using templates, you can automate the provisioning on new or existing modern SharePoint sites with your own custom configurations. Site scripts are part of site templates. Within your site script you define what kind of configuration should be done and afterwards you can combine one or multiple site scripts into a site template. Site template is what you and your users can select and apply.
Learn more about SharePoint site templates and site scripts here: SharePoint site template and site script overview | Microsoft Learn
Handling emoji in site scripts
You configured your new site script to add a new navigation link for your department or project site to make it look nicer. Here is just a small example:
{
"$schema": "schema.json",
"actions": [
{
"verb": "addNavLink",
"url": "https://support.microsoft.com",
"displayName": "📚 Support resources",
"isWebRelative": false,
"navComponent": "QuickLaunch"
}
],
"bindata": {},
"version": 1
}
I just saved this as a Json file locally on my computer.
As you can see, we added an emoji in front of the text and in Visual Studio Code or Notepad it also looks good so the icon is displayed as it should.
To easily open the Emoji Keyboard, press the windows key + period key. On a Mac Control + Command + Space
To create a new SharePoint site script, we need to use PowerShell and the SPO Management Shell as currently there is no UI to add new site scripts or site templates.
You need to be a SharePoint Administrator in your tenant to perform these actions
# Sign in
Connect-SPOService -Url "https://<your tenant>-admin.sharepoint.com"
# Get the content from the json file
$content = Get-Content -Path C:\TEMP\SiteScript.json
# Here you can see the content of the file again.
$content
{
"$schema": "schema.json",
"actions": [
{
"verb": "addNavLink",
"url": "https://support.microsoft.com",
"displayName": "📚 Support resources",
"isWebRelative": false,
"navComponent": "QuickLaunch"
}
],
"bindata": {},
"version": 1
}
Let’s just stop here for a second and review what we can already see. The emoji doesn’t really look anymore as we saw it within VS Code or Notepad before, we just see some weird stuff “📚”. The encoding isn’t proper. Let’s see if we can fix this:
# Load the content with Encoding UTF8
$content = Get-Content -Path C:\TEMP\SiteScript.json -Encoding UTF8
# Check content
$content
{
"$schema": "schema.json",
"actions": [
{
"verb": "addNavLink",
"url": "https://support.microsoft.com",
"displayName": "📚 Support resources",
"isWebRelative": false,
"navComponent": "QuickLaunch"
}
],
"bindata": {},
"version": 1
}
This looks better! Now, let’s add a new site script:
# Add new site script
Add-SPOSiteScript -Title "My fancy site script" -Content $content
# Error Message
Add-SPOSiteScript : Cannot convert 'System.Object[]' to the type 'System.String' required by parameter 'Content'.
Specified method is not supported.
At line:1 char:58
+ Add-SPOSiteScript -Title "My fancy site script" -Content $content
+ ~~~~~~~~
+ CategoryInfo : InvalidArgument: (:) [Add-SPOSiteScript], ParameterBindingException
+ FullyQualifiedErrorId : CannotConvertArgument,Microsoft.Online.SharePoint.PowerShell.AddSPOSiteScript
OK, another error. So, let’s format it into a string:
# Get content
[String]$content = Get-Content -Path C:\TEMP\SiteScript.json -Encoding UTF8
# Check the content again
$content
{ "$schema": "schema.json", "actions": [ { "verb": "addNavLink", "url": "https://support.microsoft.com", "displayName": "📚 Support resources", "isWebRelative": false, "navComponent": "QuickLaunch" } ], "bindata": {}, "version": 1 }
# Add a new site script
Add-SPOSiteScript -Title "My fancy site script" -Content $content
# output
Id : c641786d-dea4-4e9b-b43e-f3f6800401fe
Title : My fancy site script
Description :
Content :
Version : 0
IsSiteScriptPackage : False
Fantastic! Site script is created. Now let’s create a site template from this:
# Add a new site template/design
Add-SPOSiteDesign -Title "My fancy site design" -SiteScripts "c641786d-dea4-4e9b-b43e-f3f6800401fe" -WebTemplate 68
SharePoint site templates were called site designs before, you might still find this term as for example for the PowerShell command.
WebTemplate are the IDs of the SharePoint Site Templates (like Team Site or Communication Site; I know can be quite confusing…) Here are the most common:
Communication site: 68
Team Site with M365 Group: 64
Team Site: 1
Channel site: 69
Let’s check out our SharePoint communication site:
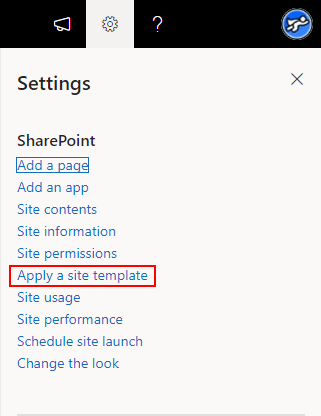
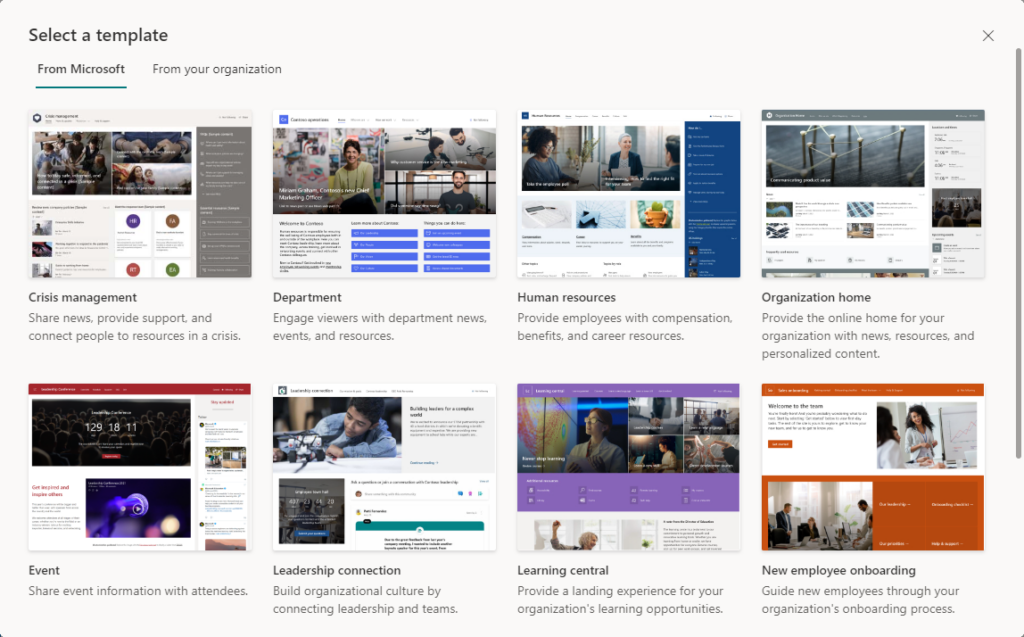
You will see some of the Microsoft default templates you can also use to apply to your site. Click on “From your organization”.
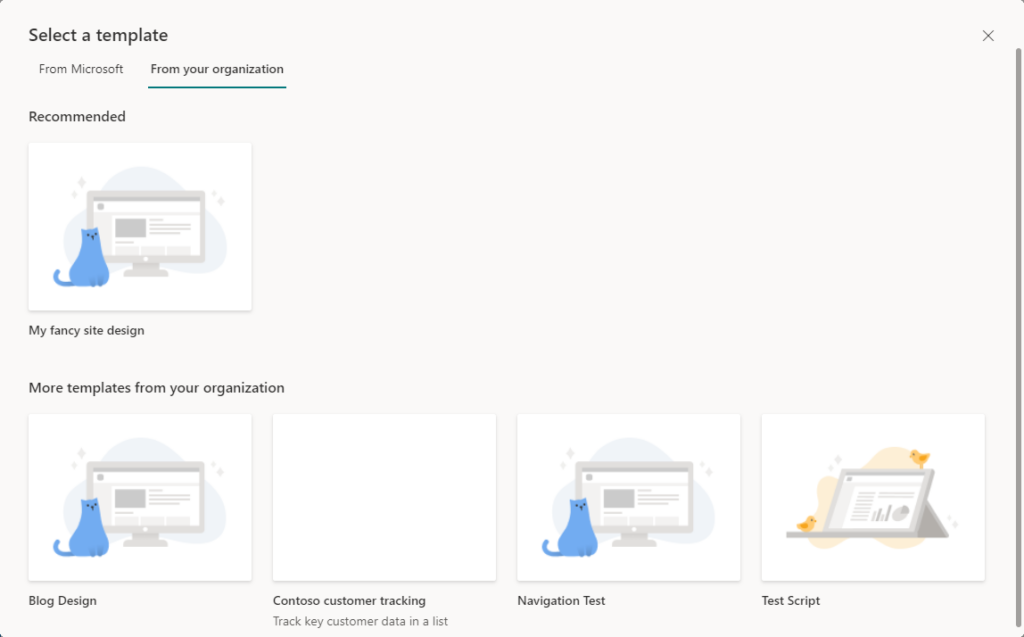
There is our newly created site template (site design). Click on it to see the details and use the template:
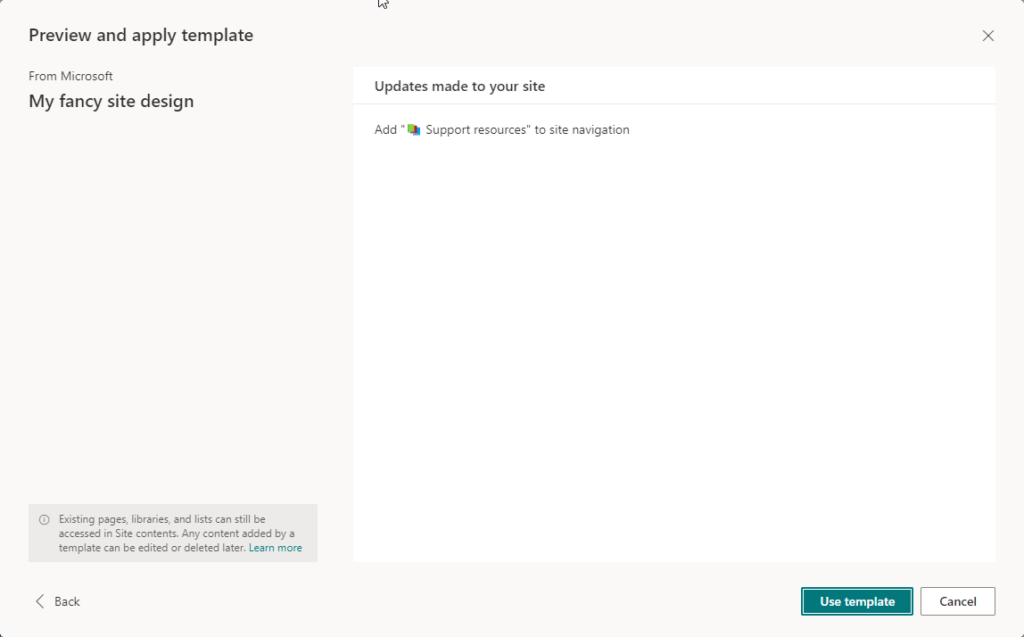
Also, our emoji looks fine! Let’s apply it.

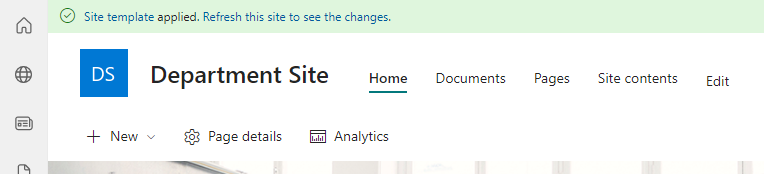

Congrats, you successfully deployed your site template, and your emoji is visible. Now let’s look at some other options.
Encode your emoji beforehand
Another option you could choose is to encode your emoji (and other characters) beforehand within your Json code:
{
"$schema": "schema.json",
"actions": [
{
"verb": "addNavLink",
"url": "https://support.microsoft.com",
"displayName": "\uD83D\uDE80 Support resources",
"isWebRelative": false,
"navComponent": "QuickLaunch"
}
],
"bindata": {},
"version": 1
}
It looks a bit weird, but in the background all emoji are like any other character just some Unicode codepoints. Just like above, we do our PowerShell:
# Load the content
$content = Get-Content -Path C:\TEMP\SiteScript.json
# Checking the content
$content
{ "$schema": "schema.json", "actions": [ { "verb": "addNavLink", "url": "https://support.microsoft.com", "displayName": "\uD83D\uDE80 Support resources", "isWebRelative": false, "navComponent": "QuickLaunch" } ], "bindata": {}, "version": 1 }
# Addind a new site script
Add-SPOSiteScript -Title "My other fancy site script" -Content $content
# output
Id : a2d9afcd-d702-4c93-bacd-d211195328c7
Title : My other fancy site script
Description :
Content :
Version : 0
IsSiteScriptPackage : False
# Add Site template/design
Add-SPOSiteDesign -Title "My other fancy site design" -SiteScripts "a2d9afcd-d702-4c93-bacd-d211195328c7" -WebTemplate 68
You can see, we didn’t need to encode our file or transform it into a string. Let’s check out the SharePoint:
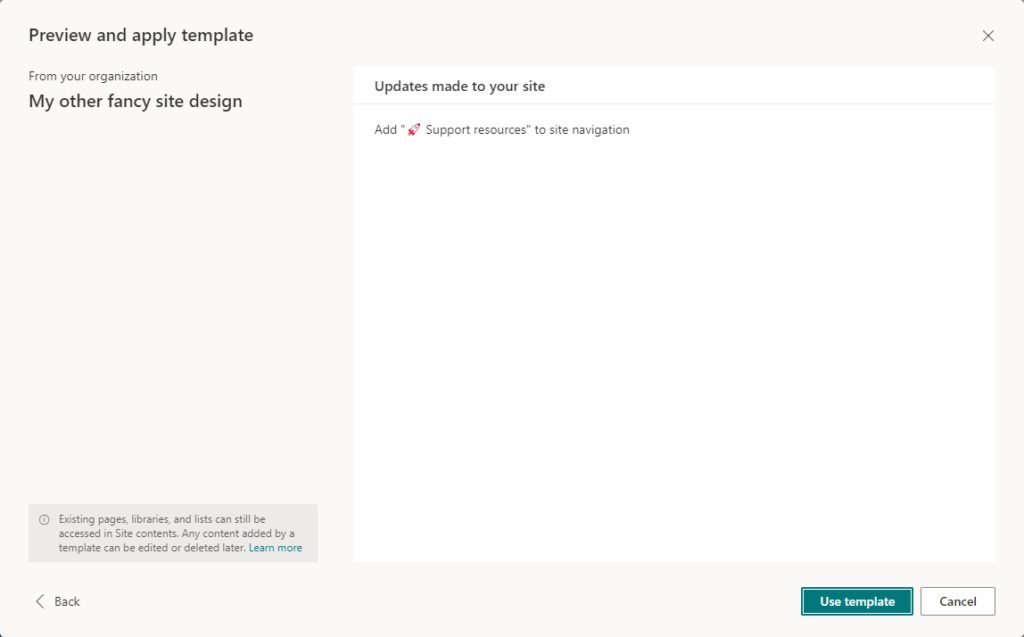
There is our other fancy site design, and you can see SharePoint properly translate the codepoint into an emoji 🚀
To find the proper Unicode codepoint, I use this site: https://unicodeplus.com/
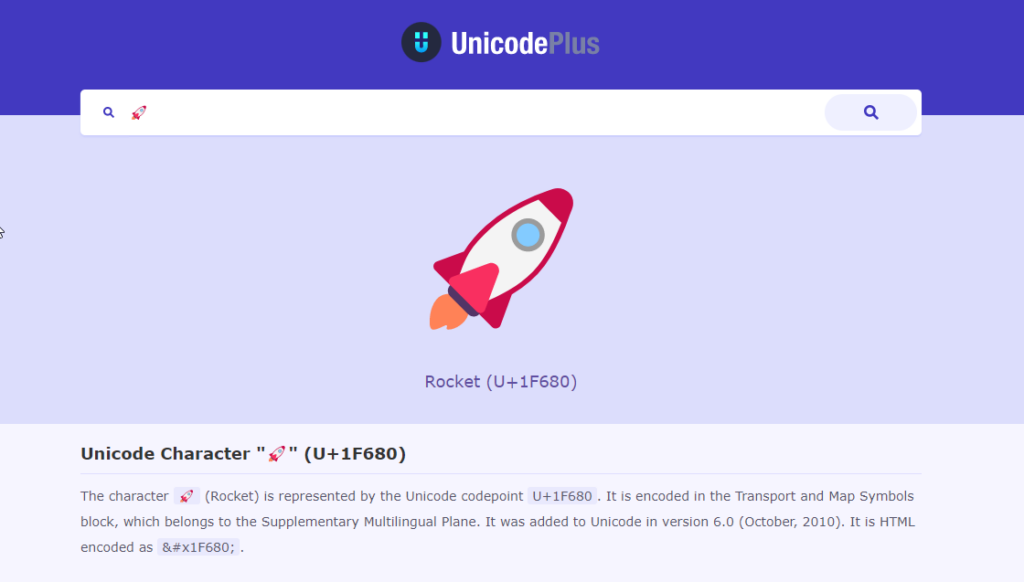
Just add your emoji into the search and you will find a lot of information about the different Unicode codepoints for the different programming languages. Scroll down to the UTF Encodings:
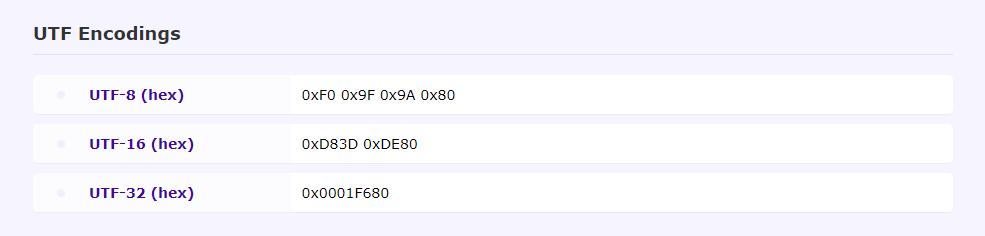
We need to use the UTF-16 (hex) encoding and still to some changes. Instead of 0x in the beginning, we need to use \u and we don’t need to space in between:
0xD83D 0xDE80 -> \uD83D\uDE80
Review
In this blog post you learned how to use emoji within site scripts to spice up your SharePoint sites. There are multiple options and the potential with site scripts and site templates to create a standardized Intranet and SharePoint environment is just at the beginning. Stay tuned for more!
Thanks for reading, I hope you liked it and it will help you!
Marvin
Schreibe einen Kommentar